mirror of
https://github.com/veekun/pokedex.git
synced 2024-08-20 18:16:34 +00:00
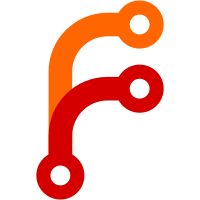
Importing pokedex can take several seconds due to its rather large dependencies—in particular, sqlalchemy, whoosh, and pkg_resources seem to be the largest offenders. Normally, it would be possible to import only the submodules one needs (pokedex.db, say), but pokedex.__init__ brings in all the submodules, for use by the command-line interface. The fix is rather obvious: - Move the command-line stuff into pokedex.main. Note: because the submodules are no longer imported by default, any script which expects `import pokedex` to be useful will likely break. Note: the `pokedex` command will not work until you re-run `python setup.py develop`, to update entry_points.txt. - Don't import pkg_resources until necessary.
47 lines
1.2 KiB
Python
47 lines
1.2 KiB
Python
""" pokedex.defaults - logic for finding default paths """
|
|
|
|
import os
|
|
|
|
def get_default_db_uri_with_origin():
|
|
uri = os.environ.get('POKEDEX_DB_ENGINE', None)
|
|
origin = 'environment'
|
|
|
|
if uri is None:
|
|
import pkg_resources
|
|
sqlite_path = pkg_resources.resource_filename('pokedex',
|
|
'data/pokedex.sqlite')
|
|
uri = 'sqlite:///' + sqlite_path
|
|
origin = 'default'
|
|
|
|
return uri, origin
|
|
|
|
def get_default_index_dir_with_origin():
|
|
index_dir = os.environ.get('POKEDEX_INDEX_DIR', None)
|
|
origin = 'environment'
|
|
|
|
if index_dir is None:
|
|
import pkg_resources
|
|
index_dir = pkg_resources.resource_filename('pokedex',
|
|
'data/whoosh-index')
|
|
origin = 'default'
|
|
|
|
return index_dir, origin
|
|
|
|
def get_default_csv_dir_with_origin():
|
|
import pkg_resources
|
|
csv_dir = pkg_resources.resource_filename('pokedex', 'data/csv')
|
|
origin = 'default'
|
|
|
|
return csv_dir, origin
|
|
|
|
|
|
def get_default_db_uri():
|
|
return get_default_db_uri_with_origin()[0]
|
|
|
|
def get_default_index_dir():
|
|
return get_default_index_dir_with_origin()[0]
|
|
|
|
def get_default_csv_dir():
|
|
return get_default_csv_dir_with_origin()[0]
|
|
|
|
|